From 6defee35c383d740022651f58c5d951b107620f9 Mon Sep 17 00:00:00 2001
From: Madison Scott-Clary We’ll be using the different methods that we outlined in the Overview above to access the available elements in the file. The easiest way to access a single element in the DOM is by its unique ID. We can grab an element by ID with the The easiest way to access a single element in the DOM is by its unique ID. You can get an element by ID with the Accessing Elements by ID
-getElementById()
method of the document
object.getElementById()
method of the document
object.document.getElementById();
We can be sure we’re accessing the correct element by changing the border
property to purple
.
You can be sure you’re accessing the correct element by changing the border
property to purple
.
```custom_prefix(>) demoId.style.border = ‘1px solid purple’;
-Once we do so, our live page will look like this:
+Once you do so, your live page will look like this:
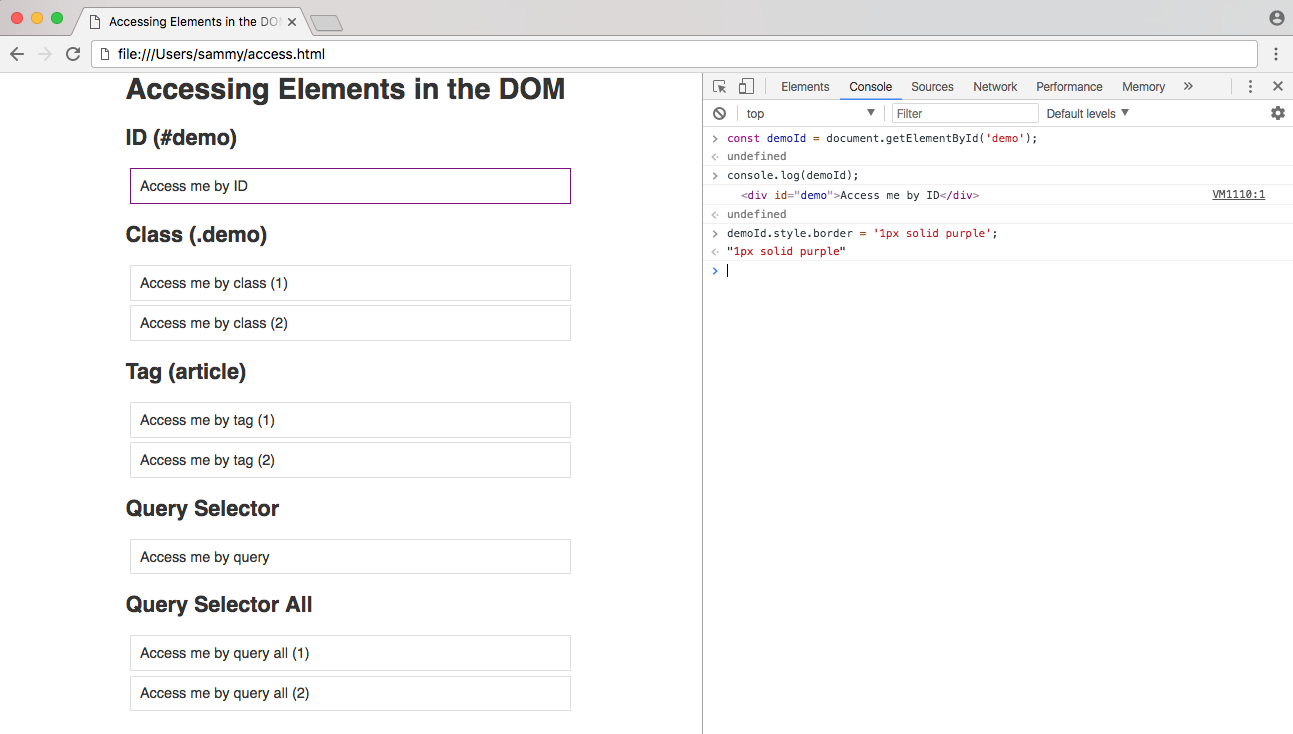
@@ -136,7 +136,7 @@ demoId.style.border = ‘1px solid purple’;
## Accessing Elements by Class
-The [class](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/class) attribute is used to access one or more specific elements in the DOM. We can get all the elements with a given class name with the [`getElementsByClassName()`](https://developer.mozilla.org/en-US/docs/Web/API/Document/getElementsByClassName) method.
+The [class](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/class) attribute is used to access one or more specific elements in the DOM. You can get all the elements with a given class name with the [`getElementsByClassName()`](https://developer.mozilla.org/en-US/docs/Web/API/Document/getElementsByClassName) method.
```js
document.getElementsByClassName();
@@ -147,10 +147,10 @@ demoId.style.border = ‘1px solid purple’;
<div class="demo">Access me by class (2)</div>
-Let’s access our elements in the Console and put them in a variable called demoClass
.
+Access these elements in the Console and put them in a variable called demoClass
.
```custom_prefix(>)
const demoClass = document.getElementsByClassName(‘demo’);
-At this point, you might think you can modify the elements the same way you did with the ID example. However, if we try to run the following code and change the `border` property of the class demo elements to orange, we will get an error.
+At this point, it might be tempting to modify the elements the same way you did with the ID example. However, if you try to run the following code and change the `border` property of the class demo elements to orange, you will get an error.
```custom_prefix(>)
demoClass.style.border = '1px solid orange';
@@ -160,7 +160,7 @@ const demoClass = document.getElementsByClassName(‘demo’);
Uncaught TypeError: Cannot set property 'border' of undefined
-The reason this doesn’t work is because instead of just getting one element, we have an array-like object of elements.
+The reason this doesn’t work is because instead of just getting one element, you have an array-like object of elements.
```custom_prefix(>)
console.log(demoClass);
@@ -168,26 +168,26 @@ console.log(demoClass);
[secondary_label Output]
(2) [div.demo, div.demo]
-[JavaScript arrays](https://www.digitalocean.com/community/tutorials/understanding-arrays-in-javascript) must be accessed with an index number. We can therefore change the first element of this array by using an index of `0`.
+[JavaScript arrays](https://www.digitalocean.com/community/tutorials/understanding-arrays-in-javascript) must be accessed with an index number. You can change the first element of this array by using an index of `0`.
```custom_prefix(>)
demoClass[0].style.border = '1px solid orange';
-Generally when accessing elements by class, we want to apply a change to all the elements in the document with that particular class, not just one. We can do this by creating a for
loop, and looping through every item in the array.
+Generally when accessing elements by class, we want to apply a change to all the elements in the document with that particular class, not just one. You can do this by creating a for
loop, and looping through every item in the array.
```custom_prefix(>)
for (i = 0; i < demoClass.length; i++) {
demoClass[i].style.border = ‘1px solid orange’;
}
-When we run this code, our live page will be rendered like this:
+When you run this code, your live page will be rendered like this:
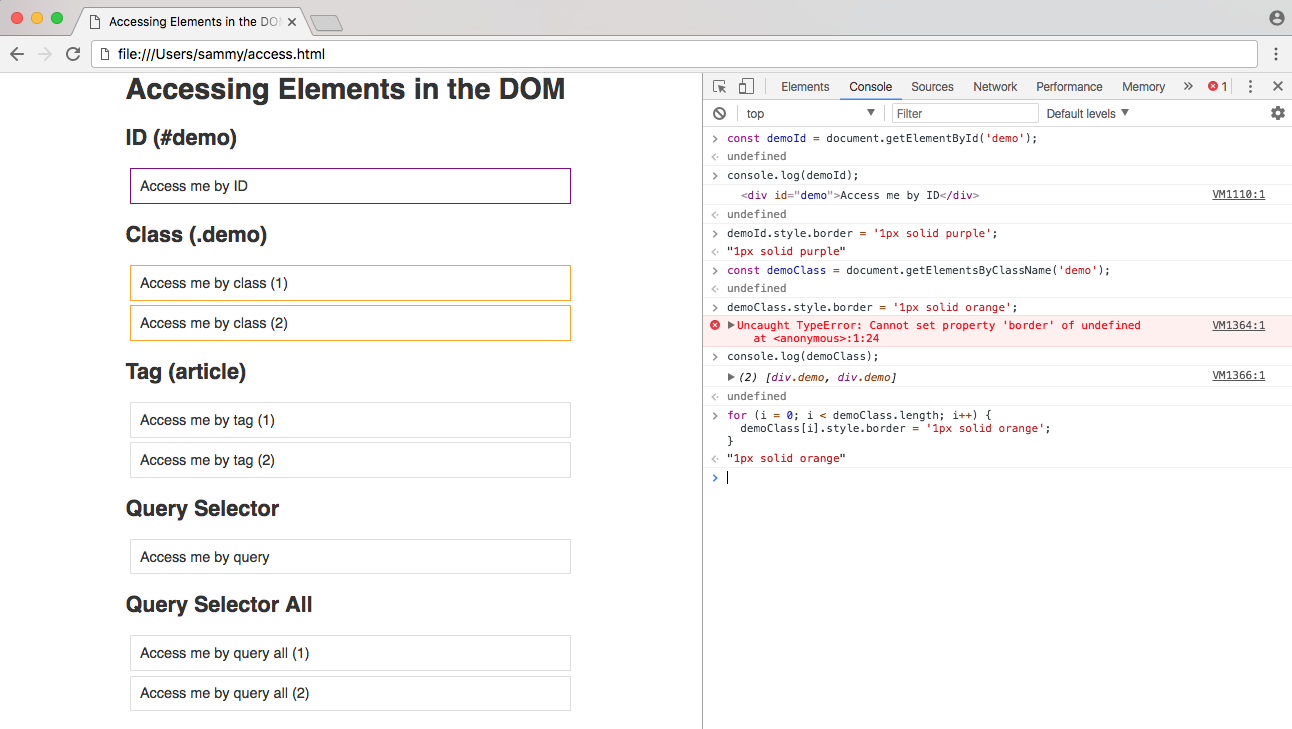
-We have now selected every element on the page that has a `demo` class, and changed the `border` property to `orange`.
+You have now selected every element on the page that has a `demo` class, and changed the `border` property to `orange`.
## Accessing Elements by Tag
-A less specific way to access multiple elements on the page would be by its HTML tag name. We access an element by tag with the [`getElementsByTagName()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/getElementsByTagName) method.
+A less specific way to access multiple elements on the page would be by its HTML tag name. You access an element by tag with the [`getElementsByTagName()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/getElementsByTagName) method.
```js
document.getElementsByTagName();
@@ -198,7 +198,7 @@ for (i = 0; i < demoClass.length; i++) {
<article>Access me by tag (2)</article>
-Just like accessing an element by its class, getElementsByTagName()
will return an array-like object of elements, and we can modify every tag in the document with a for
loop.
+Just like accessing an element by its class, getElementsByTagName()
will return an array-like object of elements, and you can modify every tag in the document with a for
loop.
```custom_prefix(>)
const demoTag = document.getElementsByTagName(‘article’);
for (i = 0; i < demoTag.length; i++) {
@@ -218,31 +218,31 @@ const demoTag = document.getElementsByTagName(‘article’);
$('#demo'); // returns the demo ID element in jQuery
-We can do the same in plain JavaScript with the querySelector()
and querySelectorAll()
methods.
+You can do the same in plain JavaScript with the querySelector()
and querySelectorAll()
methods.
document.querySelector();
document.querySelectorAll();
-To access a single element, we will use the querySelector()
method. In our HTML file, we have a demo-query
element
+To access a single element, you can use the querySelector()
method. In our HTML file, we have a demo-query
element
<div id="demo-query">Access me by query</div>
-The selector for an id
attribute is the hash symbol (#
). We can assign the element with the demo-query
id to the demoQuery
variable.
+The selector for an id
attribute is the hash symbol (#
). You can assign the element with the demo-query
id to the demoQuery
variable.
```custom_prefix(>)
const demoQuery = document.querySelector(‘#demo-query’);
-In the case of a selector with multiple elements, such as a class or a tag, `querySelector()` will return the first element that matches the query. We can use the [`querySelectorAll()`](https://developer.mozilla.org/en-US/docs/Web/API/Document/querySelectorAll) method to collect all the elements that match a specific query.
+In the case of a selector with multiple elements, such as a class or a tag, `querySelector()` will return the first element that matches the query. You can use the [`querySelectorAll()`](https://developer.mozilla.org/en-US/docs/Web/API/Document/querySelectorAll) method to collect all the elements that match a specific query.
-In our example file, we have two elements with the `demo-query-all` class applied to them.
+In the example file, you have two elements with the `demo-query-all` class applied to them.
```html
<div class="demo-query-all">Access me by query all (1)</div>
<div class="demo-query-all">Access me by query all (2)</div>
-The selector for a class
attribute is a period or full stop (.
), so we can access the class with .demo-query-all
.
+The selector for a class
attribute is a period or full stop (.
), so you can access the class with .demo-query-all
.
```custom_prefix(>)
const demoQueryAll = document.querySelectorAll(‘.demo-query-all’);
-Using the `forEach()` method, we can apply the color `green` to the `border` property of all matching elements.
+Using the `forEach()` method, you can apply the color `green` to the `border` property of all matching elements.
```custom_prefix(>)
demoQueryAll.forEach(query => {
@@ -254,7 +254,7 @@ const demoQueryAll = document.querySelectorAll(‘.demo-query-all’);
With querySelector()
, comma-separated values function as an OR operator. For example, querySelector('div, article')
will match div
or article
, whichever appears first in the document. With querySelectorAll()
, comma-separated values function as an AND operator, and querySelectorAll('div, article')
will match all div
and article
values in the document.
Using the query selector methods is extremely powerful, as you can access any element or group of elements in the DOM the same way you would in a CSS file. For a complete list of selectors, review CSS Selectors on the Mozilla Developer Network.
Complete JavaScript Code
-Below is the complete script of the work we did above. You can use it to access all the elements on our example page. Save the file as access.js
and load it in to the HTML file right before the closing body
tag.
+Below is the complete script of the work you did above. You can use it to access all the elements on our example page. Save the file as access.js
and load it in to the HTML file right before the closing body
tag.
[label access.js]
// Assign all elements
const demoId = document.getElementById('demo');
@@ -338,7 +338,7 @@ const demoQueryAll = document.querySelectorAll(‘.demo-query-all’);
In this tutorial, we went over 5 ways to access HTML elements in the DOM — by ID, by class, by HTML tag name, and by selector. The method you will use to get an element or group of elements will depend on browser support and how many elements you will be manipulating. You should now feel confident to access any HTML element in a document with JavaScript through the DOM.
+